Best Superior Porn Websites
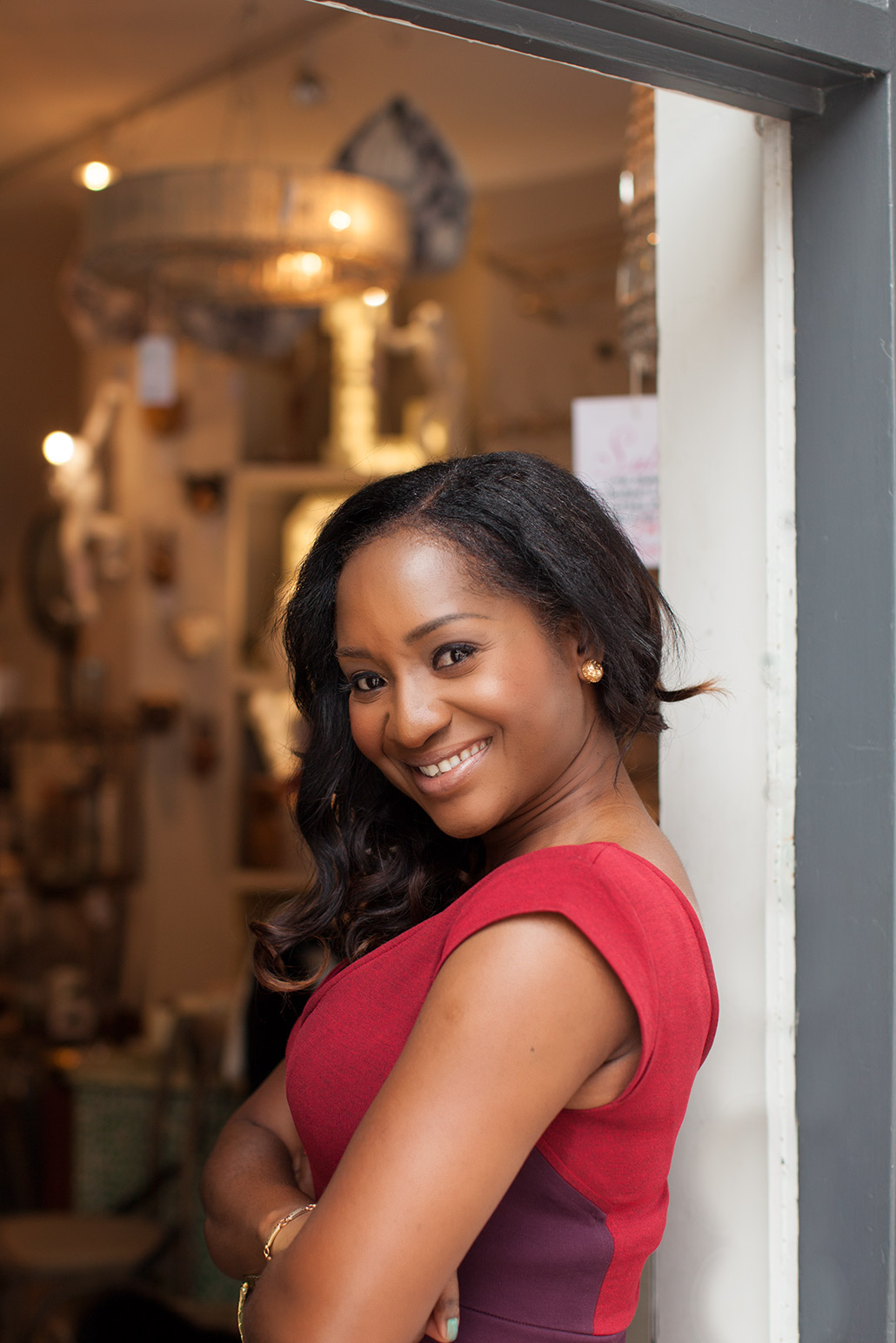
Thanks for visiting the greatest directory site of very best porn web sites and stay sexual intercourse cams. This listing will helps you to make a choice from all of the Real best porn web sites offered on the net. Our very best porn web sites list is split in numerous groups, look at your favorite to acquire a checklist of the most effective websites today. Level across the thumbnails to acquire a review for each web site.
This superior porn websites collection actually is the very best treasure from the Chart! We’ve reviewed and gathered the best porn paysites, which includes completely new sites along with well-known labels. If you are willing to pay for the best porn, here you’ll find all the premium sites that are really worth your money!
The adult industry’s greatest stored key – AdultPornList
Here at AdultPornList, we’ve devoted ourself to simply being the go-to destination for people that want to find the best porn internet sites how the Online is offering. We have dozens of categories that we’ve spent hundreds of hours researching in order to show you the top adult websites in any niche you could possibly want. Feel as if some cost-free porn video tutorials? No problem. Then why not erotic accounts? We’ve got them! Irrespective of how common or area of interest your porn wants are, you’ll have a great time undergoing all of our outlined internet sites in this article that will likely placed the most effective XXX enjoyment around proper in front of you.
To be sure the top quality of the recommendations and ratings, every single advised xxx online comic porn web site has gone through an considerable review method to make sure that our users are merely viewing the cream of the crop. For each and every 1 internet site detailed, there are 10 other people which we required a short look at which couldn’t create the grade. We’re severe with regards to very good porn: you’ll adore our choices and may wish to return everyday to see about far more amazing internet sites that have the porn you’re following. What’s more, we have all of the testimonials listed here published by experts who comprehend the particulars of adult enjoyment. They search for a variety of different characteristics in the web sites they evaluation to ensure you’re only because of the finest details achievable.
In order to try and become the go-to porn destination, it’s much easier for us to just go ahead and review destinations online that we believe are already doing a fantastic job, AdultPornList believes that instead of trying to build a website where we host all different types of content. We can focus on giving our readers the best information possible, so their exposure to our recommended top porn sites is of the highest quality, because we don’t host any content ourselves. We consider our assessment techniques really seriously and need you to recognize that every step is come to make certain only decent, operating sites are incorporated into our evaluations.
A variety of elements are considered for all of us when standing the ideal porn websites over most of these niches. These include how frequently the website upgrades, how fast web pages load, how many ads you will find, what size the archive is and so forth. The great thing for readers is that because we have dozens of categories with reviews, you can choose to enjoy live sex cams, free porn videos, adult forums, online shopping stores, torrent websites and more. You’ll shortly understand that we have a excellent vision for stellar adult websites which with regards to breaking up the excellent from the poor, couple of do it better than us. Make sure you Bookmark AdultPornList as well – doing this, you could always can come on this page later on to savor much more of our encouraged top pornographic sites!
Finest Adult Porn Sites 2023

Finest Adult Porn Internet sites: ‘Porn’ is a familiar word which often leads to controversy if it’s discussed in public. But men and women cannot management their urge to masturbate or even for gender. They require something really horny to improve up their libido. So, if you are the one feeling horny right now, we have something really special for you. This site offers you a summary of the best adult porn internet sites to setup your mood today. Look at our selection of among the best adult porn sites
How to watch Porn if it’s Banned in your country?
Perhaps you are on this post due to the fact you are trying to find a Porn web site which happens to be “not banned” within your region? Effectively. this article was previous current four years back! Consequently, the globe has remarkably converted a lot more conservative toward Porn. In many places such as India, Porn has been “censored” as with you can’t entry the internet sites but accomplishing this isn’t illegal and wouldn’t property you in prison. (Nonetheless, property or selling is stringently prohibited).
In other countries around the world, including virtually the complete Middle Eastern, Porn is straight up illegal and punishable legally. Anyway, if you’re from any country where you can’t “access” legal porn there’s a way around.
The very best porn websites categorized by niche categories and positioned by high quality
AdultPornList reveals you the greatest porn internet sites gathered into practical category details. According to our strict criteria, in order to offer a concrete list of top porn sites that we know you will like, because there’s just quality across the board, We’ve ranked them all. The ideal porn websites you might at any time find, we currently found to suit your needs!
After after an occasion there seemed to be a small grouping of porn end users that were hoping to find the most effective porn web sites. I found myself some of those folks. We started adding our favourite websites in a porn checklist, that we called Leading Porn Websites.
This is actually the porn listing we were looking for, and this is why we generate it. All the time we’ve lost looking for quality porn tubes! Alternatively, porn comics! We essential a reliable location, a safe and secure haven to enjoy simply the very best porn. At the beginning, we were looking for the very best totally free porn web sites, and we have located them, ingested them, and rated. Next, we started off with all the very best premium porn sites, as well as the online game grew to become better and better: we sensed like we had been given birth to again: we started to be paid for porn sites apologists. It was another world. Every single kind of erotic flavor from millions of porn websites. So we get a lot more total satisfaction listing our private porn superstar list: the women we love are there, right behind the display screen, on flickr, and you will communicate with them. Have to have the hyperlink? there you decide to go, you will discover it on AdultPornList.
This is basically the greatest porn list you will discover, whether or not you need to buy porn or you’re looking for the very best totally free porn websites.
Indeed, there are other porn web sites comparable to this variety, with a similar names. But ehy, we love porn and we desired our very own rating. Since a couple of things are confident about the men and women scripting this collection: we all know porn, so we enjoy porn. This is why we create the checklist. Like when you’re 12 years old and prepare the list of the girls or boys of your classroom that you like the most, or the list of the best rock bands or football players. Ranking whatever you like can be a humorous online game. So, we decided to publicly rank porn sites, it was August 2023, and we still enjoy this game: exactly where for the greatest porn? it is a never ending online game, and there is no appropriate respond to, just a thin range in between terrible porn and good porn. We understand the visible difference adequately, why don’t discuss our gift with all the social network?
Best Cost-free And Compensated Porn Websites of 2023
With new porn web sites showing up about every single area, it is hard to find the ones which can be in fact great. Effectively, AdultPornList.com eliminates that issue to suit your needs, because of so many diverse internet sites site and review information to endure, it’s not surprising we now have a lot of people visiting our website for guidance. You’ve come to the right place if you’re someone who needs suggestions about all the finest sites that you can find on the internet. If there’s one thing that AdultPornList.com does right is that it presents you with only the safest and finest porn sites that you can visit. You don’t have to worry about any of these sites scamming you if they’re premium sites, and you won’t be getting any viruses from visiting the free porn sites presented on here either. We have basically made certain that you are proceeding to have a fantastic porn expertise no matter what one of those websites you end up visiting. If you want to find out more about any one of these sites, simple hover over the name and then click on the review icon on the right side. Get ready for a experience through porn paradise which you won’t have the capacity to forget. And merely like our expertise in porn which we so happily within our website name, we can easily also assure that your safety and leisure are going to be put in initially place when you’re on our site. Start off searching AdultPornList.com and learn just why we got ourself this name.
How made it happen all get started with AdultPornList.com and why precisely did it start off anyway?
With over a decade below its belt, AdultPornList.com was began for starters cause and something cause only: to give you the finest porn websites that the world wide web provides. Why? Nicely, we all love porn, and this really is a simple fact. There is not just one individual out there that doesn’t observe any porn, so that we just desired to make certain that every person got the best time when performing this activity. It is as a consequence of our love for porn we decided to start off exhibiting you the finest sites to find the best porn on. We will highlight exactly what the porn industry has to offer, and we will assist you for the greatest options on our lists. We started all of this,. That’s the more you explore out endless lists of porn sites, the more you’ll find out exactly why it. With AdultPornList.com you can rest assured that you’ll be getting only the finest porn sites on your repertoire, though you probably already used a bunch of porn sites that are pure garbage. Continue to not certain? Properly, what about this fact that we’ve been used in excess of decade now? There are plenty of porn internet sites coming on the internet today that it’s very hard to be sure that you are making the correct and safe option, and that’s why we are in this article!
What sort of secure porn websites is it possible to expect on our website?
Let us tell you just one single issue with regards to all the web sites which can be on AdultPornList.com. You can rest assured that it’s been tried and tested and if it isn’t on our site, then it’s either a shitty site or that kind of porn just doesn’t exist anywhere, if it’s on our site. Both that or it’s unlawful. Whatever one of these motives it may be, you should not be viewing that sort of porn. Stick to AdultPornList.com and look for the safest web sites that you simply know will bring you lots of entertainment and joy. But let us end up in the nitty and gritty to get a check and bit out the specific form of websites that you could assume on in this article. Basically, expect both free and premium sites to be present on AdultPornList.com, but don’t think that you’ll be tricked that a premium site is free on here. Things such as that simply don’t take place on our site, considering that we clearly mark all of the web sites that are free of charge and all the sites which are premium and call for your hard earned money. It does not issue which form of porn you want, regardless of whether it is free or superior, you don’t need to be concerned about this not being existing on AdultPornList.com given that we’ve obtained it all.
Greatest Top quality Porn Internet sites

Good Quality Porn Internet sites to reside From The Fantasies Virtually
After keeping indoors for more than a 12 months, returning to function continues to be hard for lots of people. But right after coming back again house coming from a strenuous day at work, there is no better way to loosen up than indulging in some porn and jerking away a lot.
Locating new porn video tutorials you have not viewed before can be a every day problem. Is how superior porn websites take on this issue. They feature high quality subscriptions for their viewers. The benefits of getting a premium registration in the porn website are numerous and because of this , why a lot of people decide to buy it.
Viewing the ideal Porn with the High quality Membership
The paid for memberships of various best premium porn internet sites might include a lot of rewards from early on use of weekly up-dates to specific uploads. You can buy a membership of any of the premium porn sites and you will have access to better content immediately if you are bored of watching the same kind of porn on a daily basis. Some high quality memberships of porn websites can include the subsequent rewards:
Very early gain access to
Some top quality shell out porn internet sites supply very early entry of their information for their top quality members. This early access to new videos might be useful to you if you are bored of watching the same kind of videos. You do not have to make yourself to be excited from seeing exactly the same form of porn day-to-day. You can get new video lessons via your premium regular membership with a weekly as well as everyday.
Access to much more information
Some porn websites give their high quality participants with additional information regarding the heavens inside their porn video tutorials. If they pay for the premium membership, these websites generally have biographical information about the pornstars in a separate section which can be accessed by viewers only.
Usage of superior area
Many of these superior spend porn internet sites have got a special area of videos which are only accessible to their top quality people. This is the most tempting bit when viewers when they are deciding if they should purchase a premium membership or not.
Porn videos can get monotonous if you prefer to watch only one kind of genre. In such a case, visitors can opt to find the premium membership of porn sites hence they gain access to higher quality porn content.
Wrapping Up
Viewing porn is an profoundly pleasurable activity for many people. If the viewers buy the premium membership of the best premium porn, but it can be made even more fun. At a nominal charge, a whole new realm of raunchy attractive video tutorials awaits you. So, if you are tired of watching the same kind of porn starring the same kind of people then it is best that you choose to purchase a premium membership of any of the porn websites whose content you enjoy. It really is a worthy expenditure for most of us who take pleasure in observing porn and don’t would like to continue to keep seeing the exact same thing over and over once more.
On AdultPornList.com there exists a huge number of classes that one could pick from which will present you with a look into which porn internet site is the perfect to pick outside the group. We have a variety of very hot high quality websites that happen to be natural fire. Look into the critiques to see what’s up. Even novice websites are placed under our types in which home made sex video clips could be seen. How about reside gender camera internet sites? Webcam is broadening and you most certainly require assistance with a good choice from the appropriate webcam site. Hot image internet sites certainly are a dime twelve. Selecting the most appropriate one will help make your pic viewing pass time more pleasant. You will also find which VR or digital truth site is from the catch. Even porn game titles have grown to be more and more well-known, check that category out also. Hentai porn videos are full of anime flicks all packed with sexual activity cartoons. Very hot lesbian category is our individual beloved, so see which lesbo internet site we look at to be the best. Additionally, there are other types likeGIFs and Forums, and sexual activity accounts websites where by we level them by good quality. Proceed to the categories that you just maintain beloved and check out our choice, it’s all rather kick ass!