Very best Webcam Websites – Observe Undressed Cam Girls in Stay Cam Reveals

InspectorCams.com is a expert sexual intercourse cam review site showcasing the very best in stay grownup leisure. Our objective is to assist you to discover which are the most useful webcam web sites, and that are, well, under stellar. You know that our mission – to generate the best sex cam reviews collection the world has ever seen – is never-ending if you’ve visited our site in the past.
We have been generally working hard maintaining our testimonials current, while planning to overview new rising cam sites of top quality. And, our company is make an effort to focused on answering the questions our viewers have about cam internet sites, through the imprecise concerns to the more standard, including the least expensive cam websites as well as the most popular gender cam marketing promotions.
Trying to find on the internet Webcam website with total strangers? InspectorCams.com gives a summary of finest graded reside webcam sites on the web. Our self-sufficient testimonials display the differences in between each webcam site and supply details that is vital to know prior to signing up. We have tested for best movie and mp3 top quality as well as cam velocity for each webcam web site. We simply level websites without any concealed service fees with out sneaky unexpected situations. Websites that made our top listings work most effectively and a lot well-known cam internet sites divided by types of webcams.
How Do We Ranking the Top Scored Webcam Sites?
We ranked the best adult webcam sites according to a wide range of criteria. We deal with the number of webcam versions each and every internet site has, precisely what the design selection is similar to, and how good your camera quality is on every single website. We also assess the support service at each internet site, along with the easy navigating and ultizing each and every website. Of course, we also talk about prices around the xxx conversation internet sites and the greatest marketing promotions to get the gay porn live cam best value to your buck.
In most cases, the principle requirement we give attention to although performing our cam web site testimonials are: How well does a cam site functionality using its attribute products, what cam present types are offered, which repayment methods can be purchased, and which varieties of types and fetishes is available on every cam web site.
How Can We Position the most notable Rated Webcam Websites?
According to a wide range of criteria we ranked the best adult webcam sites. We deal with how many webcam types each web site has, just what the version assortment is similar to, and the way excellent the camera quality is on every site. We also review the customer service at every site, plus the comfort of navigating and making use of each and every site. Obviously, we talk about prices in the xxx chitchat web sites and the greatest promotions for the greatest value for your dollar.
Generally speaking, the key requirement we give attention to while undertaking our cam web site critiques are: How good does a cam site function using its function products, what cam show varieties are offered, which repayment strategies can be purchased, and which types of types and fetishes can be found on every cam site.
XXX Cams for every single Taste and Fetish
Our selection of the best scored webcam websites will give you the chance to choose a site according the criteria that issues most for you. Whether it be cost, quantity of cam women, display screen image resolution, vacation campaigns, sexual activity conversation discount rates, totally free webcam credits, or some other attribute that’s vital that you you, Very best Webcam Sites provides all of you the data.
We’re well aware of the reality that just what the regular established through the mainstream media is far from becoming the majority’s ideal. Our company is really as diverse since they arrive in terms of erotic likes and dislikes, and this is the reason we absolutely fully grasp and regard every lawful erotic preference.
Until now, we’ve covered the most common and requested sex chat categories, but we intend to go much further. We’re ceaselessly hunting for specialized and interesting mature conversation web sites about which we could write. Here’s several examples of whatever we have to date:
- Very best Older Cam Sites
- Best Oriental Conversation Web sites
- Best Ebony Webcams
- Ft . Cam Websites
- Greatest Gay Video clip Chitchat
- Shemale Chitchat Web sites
- Latina Cam Websites
- BBW Sex Cams
Or just don’t really want to do much more reading, our top recommendation is Camsoda, if you are unsure of where to go. They have a wonderful product variety, every fetish you can imagine, as well as the cam2cam function is within the cost of a individual chitchat. It’s tough to fail using this type ofinnovative and affordable, and trusted webcam website,
Try out Online Sex Cams Websites
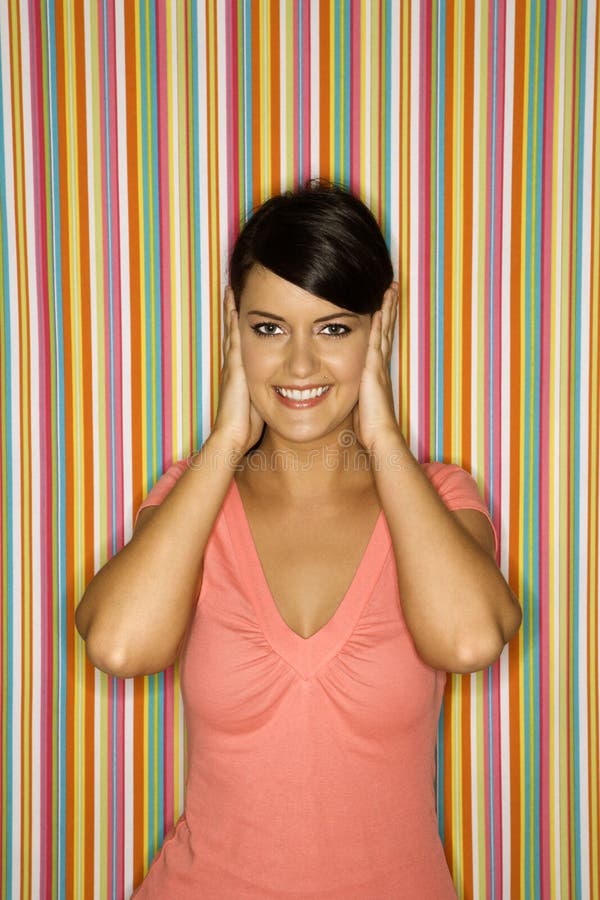
If you are tired of the same old cam sites where the girls are paid to be there, and you are just another number with a dollar sign, you have come to the right place! Gender cams is undoubtedly an adult webcam community exactly where standard folks from all over the world go toflirt and meet, and possess totally free webcam talk! Sexual intercourse Cams is 100% able to be a part of, without any credit card required to turn into a member. You can fulfill folks randomly and open up a arbitrary Sexual intercourse webcam in a number of our concept structured webcam areas.
You have to be a minimum of 18 years to sign up with and make use of this particular service, as it is a totally free mature webcam website. You can easily become a free member by completing our quick account registration form, though you do not even need to register to enter our Sex chat rooms as a guest. If you do become a free member, there are no monthly fees of any kind, and no credit card is required for membership!
Evaluations OF 2023’S Greatest LIVE Talk SITES
Familiarize yourself with the very best webcam sites on-line by studying our critiques. On this page, you’ll locate a good amount of information on personal cam-to-cam costs, great talk space features, and promotions at all of the greatest livestreaming internet sites. Our comparison and reviews posts aim to keep followers of webcam displays knowledgeable and able to engage. So, please have a look through our reputable reviews and choose a site that is perfect for your needs and budget.
WHAT Specifies THE BEST Video clip Talk Web sites?
Many users say that they’re looking for the best webcam site online, but not everyone know what that means. Just when was a webcam talk group is recognized as genuinely extraordinary and once would it be as being a 50 other people? You will find a number of criteria which you ought to look for in any high quality cam livewebcam website: Price ranges have to be affordable, there ought to be a good solution of camporn chicks, the livesex chitchat place features need to operate easily, as well as the internet streaming top quality needs to be great. There is no reason to give up any of these aspects, because you, as a user, deserve a high-quality, legit livecams experience when you pay for it. The best live porno videochat sites do offer all of these things, but there are also many web-sites that charge a high price and don’t deliver.
LIVE WEBCAM Evaluations ARE A Great Way To Obtain Information and facts
There are still users that end up paying more than they should and getting less than they deserve, because it’s difficult for one person to spend time on every chat community on the web and make comparisons with an experienced, expert eye. Very best Stay Cam Websites is available with the necessary details. Our research squads compare, collect and update, and you can start using these reviews to get every one of the facts about bestcamgirls along with the web sites they hail from, when you will need them, making wiser and a lot more informed choices about ranked and rated sexcam web sites.
SAVE MONEY By Utilizing The Greatest Stay CAM Marketing promotions
A lot of the greatest stay pornsites online are providing some form of a particular marketing that customers can take advantage of. These deals range from added bonus credits to totally free celebrity demonstrates and even free warm women-on-cam chats. And several camlive web sites change their marketing promotions on a regular basis to hold point interesting. On BestLiveCamSites.com we continue to keep examining the best portals for new gives, so you can take pleasure in the lowest priced rates for the finest girls about. Read the in depth evaluations listed for your personal favored webcamslive websites, and who is familiar with, You will probably find out that you’ve been missing out on an incredible camchat provide.
What You are likely to Get out of Top 5 Cam Sites
Following the time, you, like me, want the very best value for your money while keeping yourself anonymous, secure and confident that the interest will stay simply that, and that nobody undesirable will stick their nostrils with your enterprise.
To put it simply, Top 5 Cam Websites will save you time searching for what’s most effective for you. I will help point you in the direction of the most dependable and many trustworthy cam sites, to help you stay away from spending money on subpar substitutes. Cam porn is a very competing sector; there are many sexual activity chat internet sites on the market, so expertise is crucial.
With my cam website critiques, I am just looking to provide a more all-all around image of the internet site; conveying the things i am looking for, what capabilities I might like in comparison to the characteristics the site offers. Also i verify how clean the actual site is, and exactly how the stay cam versions connect with the two free of charge and paying out consumers. Above all, I’ll explain to you candidly about the quality of the site’s types, shows and important features like cam2cam.
With my blog sites, I’ll be generating an endeavor to tell you of the greatest adult talk websites in specific categories, like the top secure cam internet sites, most subtle cam2cam websites as well as the best grown-up cam web sites that agree to gift certificates. My objective is to experience a website for every single niche and query, in order that not one people will abandon Top Five Cam Sites with inquiries unanswered or wishes disappointed.
All in all, you’ll be able to find whatever floats your boat, check if the live adult cam site offering it is any good and get informed about is weaknesses, the and strengths myriad of ways you could be saving money on it.
How InspectorCams.com Select These webcam web sites
InspectorCams choose all those webcam internet sites in original and smart method. InspectorCams actually tests every webcam site for few days before writing a review, rather than most of other webcam site lists do. Most end users simply check a webcam web site for several minutes or so and after that compose evaluation regarding this. InspectorCams in their change takes enough time being as lively members; our team can make close friends, webcam web sites with others and gives every single come with a go. Our team could honestly say that InspectorCams brings user the most qualitative adult webcam sites, by doing this.
As soon as we has examined a webcam internet site for a few days, just analyze each feature, we compose an entire assessment about it and ranking it amongst every one of the other mature webcam websites.
Our listing of top rated grown-up webcam websites features just the greatest grownup only webcam websites online. End user would study some critiques of each and every webcam internet site see important info about each and every webcam website plus understand how many people typically use every webcam internet site. InspectorCams really do get complete work out of finding a very best adult webcam site on the internet.
Tips
- Before chatting.
- Has to be above 18 years old to work with the site and watch Grownup website cam women
- Maintain your private information personal. Expressing standard facts are fine but details are not essential.
- Have some fun and become respectful.
Make sure you chose the category of Adult girl you want to get acquainted with,
Differing People, Distinct Choices
Sex can be a beautiful and bizarre rainbow of fetishes, kinks and preferences, which is consequently extremely subjective. This is why I find it so imperative to stay objective. I’m will be referring to online video high quality, choice of versions and demonstrates, rates, customer support and the like. Items you can accurately grade and examine. That’s also why I talk about everything and anything.
I have got experienced the hassle of reviewing basically all types of adultcam site imaginable. For you personally enthusiasts of older ladies, I suggest looking into my listing of recommended adult cam sites. But are having cravings for girl on girl action, check out our list of sites with the best lesbian cam girls, if you aren’t looking for older. If you prefer chicks with dicks, hop on over to the reviews of the best shemale cam sites I meticulously created.
No matter what becomes you away from, you’ll find Top 5 Cam Internet sites as a beneficial manual and resource.